API DOCUMENTATION
Your Guide to GTIPAY API Implementation
This section describes the GTIPAY payment gateway API.
Introduction
GTiPAY API is easy to implement in your business software. Our API is well formatted URLs,
accepts cURL requests, returns JSON responses.
You can use the API in test mode, which does not affect your live data.
The API key is use to authenticate the request and determines the request is valid payment or not.
For test mode just use the sandbox URL and In case of live mode use the live URL from section Initiate Payment.
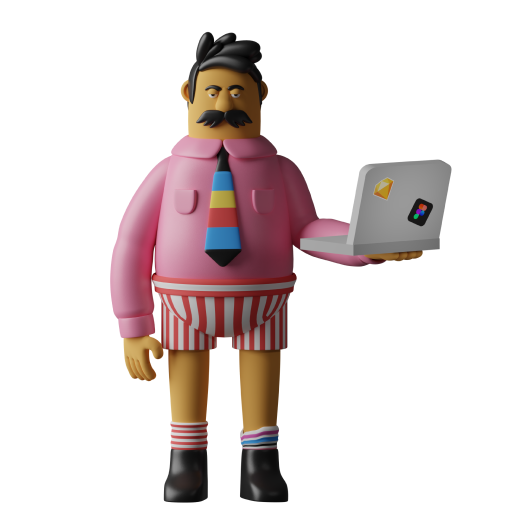
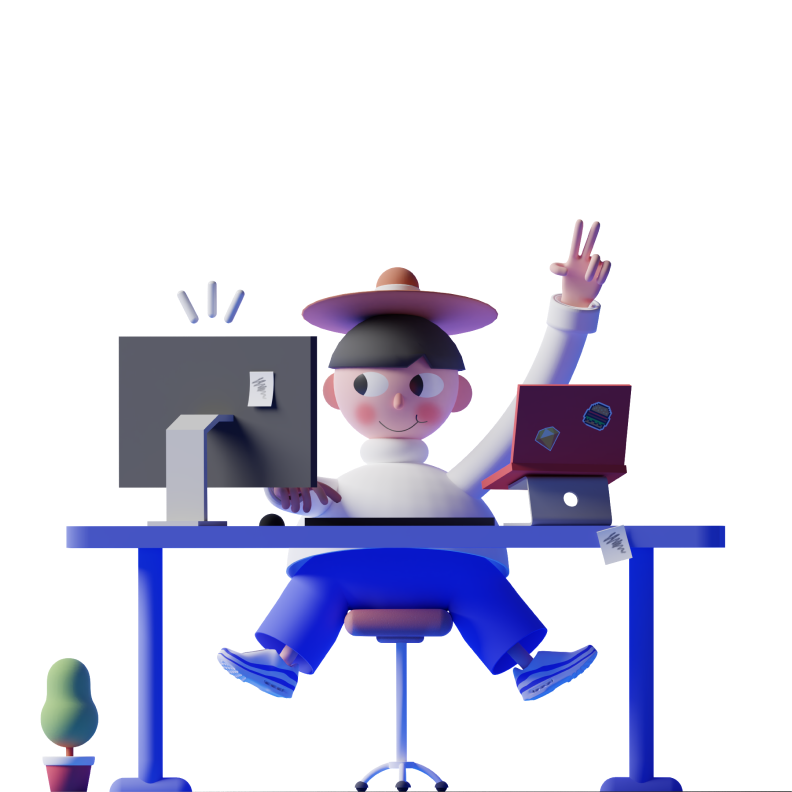
Get The Api Key
This section describes how you can get your api key.
Login to your GTiPAY merchant account. If you don't have any? Click Here
Next step is to find the Api Key menu in your dashboard sidebar. Click the menu.
The api keys can be found there which is Public key and Secret key.
Use these keys to initiate the API request.
Every time you can generate new API key by clicking Generate Api Key button.
Remember do not share these keys with anyone.
Initiate Payment
This section describes the process of initaiing the payment.
To initiate the payment follow the example code and be careful with the perameters.
You will need to make request with these following API end points.'
Live End Point: https://gtipay.com/payment/initiate
Test End Point: https://gtipay.com/sandbox/payment/initiate
Test Mode Mail:' test_mode@mail.com
Test Mode Verification Code: 222666
Request Method:' POST
Request to the end point with the following parameters below.
Param Name | Param Type | Description |
---|---|---|
public_key | string (50) | Required Your Public API key |
identifier | string (20) | Required Identifier is basically for identify payment at your end |
currency | string (4) | Required Currency Code, Must be in Upper Case. e.g. USD,EUR |
amount | decimal | Required Payment amount. |
details | string (100) | Required Details of your payment or transaction. |
ipn_url | string | Required The url of instant payment notification. |
success_url | string | Required Payment success redirect url. |
cancel_url | string | Required Payment cancel redirect url. |
site_logo | string/url | Required Your business site logo. |
checkout_theme | string | Optional Checkout form theme dark/light. |
customer_name | string (30) | Required Customer name. |
customer_email | string (30) | Required Customer valid email. |
$parameters = [
'identifier' => 'DFU80XZIKS',
'currency' => 'USD',
'amount' => 100.00,
'details' => 'Purchase T-shirt',
'ipn_url' => 'http://example.com/ipn_url.php',
'cancel_url' => 'http://example.com/cancel_url.php',
'success_url' => 'http://example.com/success_url.php',
'public_key' => 'jkUiYUS9875VDLFJLDSAFJLDKSisad',
'site_logo' => 'http://example.com/logo_path/logo.png',
'checkout_theme' => 'dark',
'customer_name' => 'John Doe',
'customer_email' => 'john@mail.com',
]
//live end point
$url = 'http://example.com/payment/initiate';
//test end point
$url = 'http://example.com/sandbox/payment/initiate';
$ch = curl_init();
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, false);
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_POSTFIELDS, $parameters);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$result = curl_exec($ch);
curl_close($ch);
//$result contains the response back.
//Error Response.
{
"error": "true",
"message": "Invalid api key"
}
//Success Response.
{
"success": "ok",
"message": "Payment Initiated. Redirect to url.",
"url":"http://example.com/initiate/payment/checkout?payment_id=eJSAASDxdrt4DASDASVNASJA7893232432cvmdsamnvASF"
}
Validate The Payment and IPN
This section describes the process to get your instant payment notification.
To initiate the payment follow the example code and be careful with the perameters. You will need to make request with these following API end points.
End Point: Your business application ipn url.
Request Method: POST
You will get following parameters below.
Param Name | Description |
---|---|
status | Payment success status. |
identifier | Identifier is basically for identify payment at your end. |
signature | A hash signature to verify your payment at your end. |
data | Data contains some basic information with charges, amount, currency, payment transaction id etc. |
//Receive the response parameter
$status = $_POST['status'];
$signature = $_POST['signature'];
$identifier = $_POST['identifier'];
$data = $_POST['data'];
// Generate your signature
$customKey = $data['amount'].$identifier;
$secret = 'YOUR_SECRET_KEY';
$mySignature = strtoupper(hash_hmac('sha256', $customKey , $secret));
$myIdentifier = 'YOUR_GIVEN_IDENTIFIER';
if($status == "success" && $signature == $mySignature && $identifier == $myIdentifier){
//your operation logic
}
Payment Terminal
This section describes the payment terminal and how to use its API.
Generate Transaction ID
To generate transaction ID for recurrent or one time transaction
End Point: /4500/api/terminal/createtrx
Request Method: POST
Send the following parameters below.
Param Name | Description |
---|---|
Merchant email. | |
amount | Amount to charge. |
description | Description to attach to payment. |
data (Optional) | Data contains some basic information with charges, amount, currency, payment transaction id etc. |
Header Param | Description |
---|---|
Authorization | Merchant Public API |
You will get following parameters below.
Param Name | Description |
---|---|
status | Payment success status. |
transaction | This contains all details of the transaction which includes user id, user type, wallet id, currency id amount, transaction charges and details. |
$url = https://gtipay.com/api/terminal/createtrx;
$fields = [
"email" => "merchantemail@email.com",
"amount" => 53.10,
"description" => "Payment for a branded T-shirt"
];
$fields_string = http_build_query($fields);
//open connection
$ch = curl_init();
//set the url, number of POST vars, POST data
curl_setopt($ch,CURLOPT_URL, $url);
curl_setopt($ch,CURLOPT_POST, true);
curl_setopt($ch,CURLOPT_POSTFIELDS, $fields_string);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
"Authorization: Bearer MERCHANT_PUBLIC_KEY",
"Cache-Control: no-cache",
));
//So that curl_exec returns the contents of the cURL; rather than echoing it
curl_setopt($ch,CURLOPT_RETURNTRANSFER, true);
//execute post
$result = curl_exec($ch);
echo $result;
}
//Error Response.
{
"error": "Merchant does not exist",
}
//Success Response.
{ "transaction":
{
"user_id":2,
"user_type":"MERCHANT",
"wallet_id":null,
"currency_id":1,
"method_code":"404",
"method_currency":"USD",
"amount":"230",
"charge":4.3,
"detail":"1234567",
"rate":1,
"final_amo":234.3,
"btc_amo":0,
"btc_wallet":"",
"trx":"OMT9JE4TOPQG",
"try":0,
"status":0,
"updated_at":"2023-02-18T20:52:03.000000Z",
"created_at":"2023-02-18T20:52:03.000000Z",
"id":57
}}
Send OTP
To verify that the user is the card owner.
End Point: /api/terminal/sendotp
Request Method: POST
Send the following parameters below.
Param Name | Description |
---|---|
Merchant email. | |
amount | Amount to charge. |
description | Description to attach to payment. |
data | Data contains some basic information with charges, amount, currency, payment transaction id etc. |
Header Param | Description |
---|---|
Authorization | Merchant Public API |
You will get following parameters below.
Param Name | Description |
---|---|
response | Response to indicate if otp was sent succesfully or not. |
$url = https://gtipay.com/api/terminal/sendotp;
$fields = [
"cvv" => 230,
"expiry" => "01/2023",
"number" => "1234567",
"trxid" => "OQDZN79S6J3K"
];
$fields_string = http_build_query($fields);
//open connection
$ch = curl_init();
//set the url, number of POST vars, POST data
curl_setopt($ch,CURLOPT_URL, $url);
curl_setopt($ch,CURLOPT_POST, true);
curl_setopt($ch,CURLOPT_POSTFIELDS, $fields_string);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
"Authorization: Bearer MERCHANT_PUBLIC_KEY",
"Cache-Control: no-cache",
));
//So that curl_exec returns the contents of the cURL; rather than echoing it
curl_setopt($ch,CURLOPT_RETURNTRANSFER, true);
//execute post
$result = curl_exec($ch);
echo $result;
}
//Error Response.
{
"error": "One or more card details are incorrect.",
}
//Success Response.
{
"response":"OTP sent to card owner.",
}
Process Transaction
To process or finish a transaction.
End Point: /api/terminal/process
Request Method: POST
Send the following parameters below.
Param Name | Description |
---|---|
cvv | Card cvv. |
expiry | Card expiry date (mm/yyyy). |
number | Card number. |
trxid | Data contains some basic information with charges, amount, currency, payment transaction id etc. |
otp | One time password (OTP) sent to card owner. |
Header Param | Description |
---|---|
Authorization | Merchant Public API |
You will get following parameters below.
Param Name | Description |
---|---|
response | Response to indicate if transaction was successful or not. |
$url = https://gtipay.com/api/terminal/process;
$fields = [
"cvv":230,
"expiry" => "01/2023",
"number" => "1234567",
"trxid" => "OQDZN79S6J3K",
"otp" => 251769
];
$fields_string = http_build_query($fields);
//open connection
$ch = curl_init();
//set the url, number of POST vars, POST data
curl_setopt($ch,CURLOPT_URL, $url);
curl_setopt($ch,CURLOPT_POST, true);
curl_setopt($ch,CURLOPT_POSTFIELDS, $fields_string);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
"Authorization: Bearer MERCHANT_PUBLIC_KEY",
"Cache-Control: no-cache",
));
//So that curl_exec returns the contents of the cURL; rather than echoing it
curl_setopt($ch,CURLOPT_RETURNTRANSFER, true);
//execute post
$result = curl_exec($ch);
echo $result;
}
//Error Response.
{
"error": "Otp did not match",
}
//Success Response.
{
"response":"Transaction completed."
}
Reverse Transaction
To reverse a payment. Merchants can refund a payment to a user. Transaction charges from the reversed transaction are also refunded to the user.
End Point: /api/terminal/reverse/{transaction-id}
Request Method: GET
Send the following parameters below.
Header Param | Description |
---|---|
Authorization | Merchant Public API |
You will get following parameters below if reversal is successful.
Param Name | Description |
---|---|
success | Message indicating that request was successful. |
You will get following parameters below if reversal fails.
Param Name | Description |
---|---|
error | Reason for request failure. |
$url = https://gtipay.com/api/terminal/reverse/ZB9J436YV6OB;
$fields = [
];
$fields_string = http_build_query($fields);
//open connection
$ch = curl_init();
//set the url, number of POST vars, POST data
curl_setopt($ch,CURLOPT_URL, $url);
curl_setopt($ch,CURLOPT_POST, true);
curl_setopt($ch,CURLOPT_POSTFIELDS, $fields_string);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
"Authorization: Bearer MERCHANT_PUBLIC_KEY",
"Cache-Control: no-cache",
));
//So that curl_exec returns the contents of the cURL; rather than echoing it
curl_setopt($ch,CURLOPT_RETURNTRANSFER, true);
//execute post
$result = curl_exec($ch);
echo $result;
}
//Error Response.
{
"success" : "Transaction already reversed before. You can't do that again.",
}
//Success Response.
{ "transaction":
{
"success":"Payment reversed successfully",
}